Product:
Planning Analytics 2.0.9.x TM1_version=TM1-AW64-ML-RTM-11.0.913.10-0
Microsoft Windows 2019 server
Python 3.11
Issue:
How tell a outside application that a TM1 TI process is finished?
Suggested solution:
Use TM1PY and a python script that checks a cube, where you write when the process have finished.
Create a cube for the flags
You have dimension FlagDimension and FlagValue, as shown above.
Create a TI process that you call from the epilog in your other process, to create the value in the cube. Looks like this:
When above TI process is run the value in the cell will increase with ONE, then you can see if the process has run more than once.
You have to come up with the pFlagName for each process, and use the same name in the Python code.
Install Python, and tm1py. https://code.cubewise.com/blog/installing-tm1py/
Create a python script similar to below ( you need to change values to point to your tm1 server instance ):
# import the modules needed import os from TM1py.Services import TM1Service # Parameters for connection - python is case sensitive USER = "admin" PWD = "apple" namespace = "" ADDRESS = "192.168.50.190" gateway = "" PORT = 12354 SSL = True #Connect to the TM1 instance tm1 = TM1Service(address=ADDRESS, port=PORT, user=USER, password=PWD, ssl=SSL) # where to check in cube cube_name = 'CubeOfFlags' elementname1 = "FlagName" elementname2 = "FValue" elementstr = elementname1 + "," + elementname2 values2 = tm1.cubes.cells.get_value (cube_name=cube_name, elements = elementstr, element_separator = ',') # only to debug the code - it will show the value in terminal window print(values2) # in our example we use a bat file to run # you need to use \\ in paths with python testfilename = "c:\\temp\\testrun.cmd" # execute the process if larger than zero if values2 != None : if values2 > 0 : print ("this is more than zero") # get the process to run os.system (testfilename) # set the value to zero in the cube # so we do not run this process again # cellset to store the new data cellset = {} # Populate cellset with coordinates and value cellset[( elementname1 , elementname2 )] = 0 # send the cellset to TM1 tm1.cubes.cells.write_values( cube_name , cellset) # end of code
The line indent is important in python, that tells what code to run when the if statement is true.
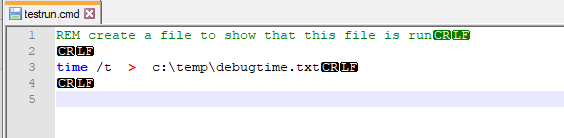
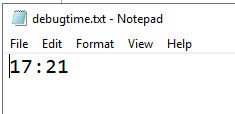
If you use the command
time /T
that will print the time. (without the /T, it will try to set the time)
str = None, element_separator: str = ‘,’, hierarchy_separator: str = ‘&&’, hierarchy_element_separator: str = ‘::’, **kwargs) → str | float
Returns cube value from specified coordinates
Parameters
• cube_name – Name of the cube
• elements – Describes the Dimension-Hierarchy-Element arrangement – Example: “Hierarchy1::Element1 && Hierarchy2::Element4, Element9, Element2” – Dimensions are not specified! They are derived from the position. – The , separates the element-selections
• dimensions – List of dimension names in correct order
• sandbox_name – str
• element_separator – Alternative separator for the element selections
• hierarchy_separator – Alternative separator for multiple hierarchies
• hierarchy_element_separator – Alternative separator between hierarchy name and
element name
Write value into cube at specified coordinates
Parameters
• value – the actual value
• cube_name – name of the target cube
• element_tuple – target coordinates
• dimensions – optional. Dimension names in their natural order. Will speed up the execution!
• sandbox_name – str
https://www.tutorialspoint.com/How-to-declare-a-variable-in-Python-without-assigning-a-value-to-it
https://code.cubewise.com/blog/loading-data-into-a-tm1-cube-with-tm1py/
https://www.learnpython.org/en/Dictionaries
https://diveintopython.org/learn/file-handling/zip
https://exploringtm1.com/cellincrementn-tm1-function-use-syntax/